Welcome to Python Get Files In Directory Tutorial. In this post, you will learn how to get files in directory using python. So let’s gets started this tutorial.
Python has various module such as os, os.path, shutil, pathlib etc by using which we can get files in directory. We will see how to work with these modules to get files.
Contents
Python Get Files In Directory – Getting Files With OS Module
In this section you will see how can you get files using OS module.
Directory Listing
OS module has two functions, by using which you can list your files.
- os.listdir( )
- os.scandir( )
os.listdir( )
- os.listdir( ) is function of directory listing used in versions of Python prior to Python 3.
- It returns a list containing the names of the entries in the directory given by path.
Let’s see an example of os.listdir( ) function. So write the following program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import os # specify your path of directory path = r"C:\Users\saba\Documents" # call listdir() method # path is a directory of which you want to list directories = os.listdir( path ) # This would print all the files and directories for file in directories: print(file) |
Now let’s check the output, this will list all the files which are present in the specified directory.
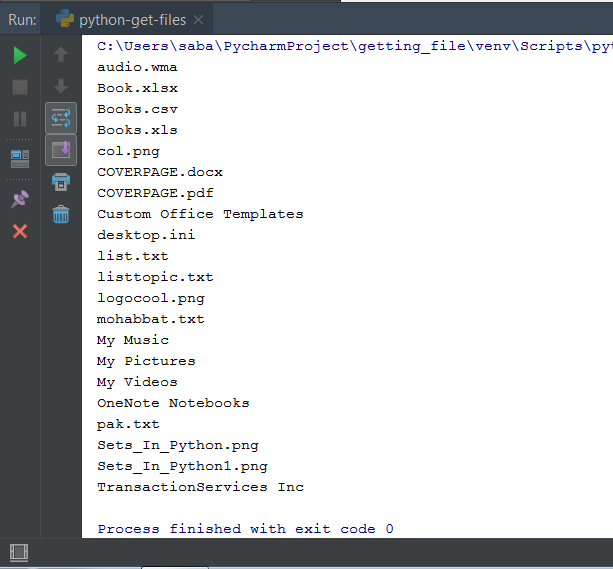
You can see all the files which are in document folder has been listed.
os.scandir( )
- It is a better and faster directory iterator.
- scandir( ) calls the operating system’s directory iteration system calls to get the names of the files in the given path.
- It returns a generator instead of a list, so that scandir acts as a true iterator instead of returning the full list immediately.
- scandir( ) was introduced in python 3.5 .
Let’s see an example of os.scandir( ), so write the following code.
1 2 3 4 5 6 7 8 |
import os # Open a file path = r"C:\Users\saba\Documents" directories = os.scandir(path) print(directories) |
The output of the above code is following –
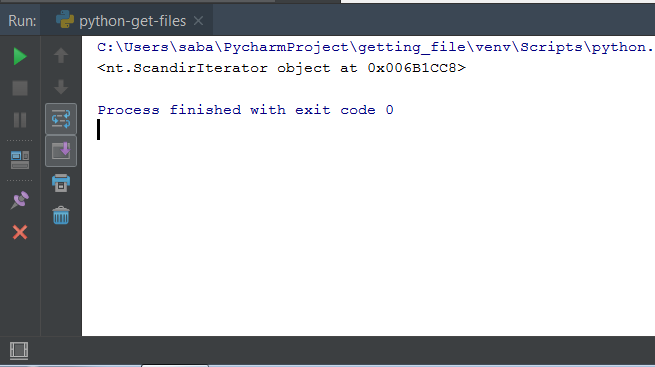
The ScandirIterator points to all the entries in the current directory.
If you want to print filenames then write the following code.
1 2 3 4 5 6 7 8 9 10 |
import os # Open a file path = r"C:\Users\saba\Documents" with os.scandir(path) as dirs: for entry in dirs: print(entry.name) |
This will give you output as below.
Listing All Files In A Directory
Now you have to list all files in a directory that means printing names of files in a directory. So let’s write the following code.
1 2 3 4 5 6 7 8 9 10 |
import os # Open a file path = r"C:\Users\saba\Documents" for files in os.listdir(path): if os.path.isfile(os.path.join(path, files)): print(files) |
- When os.listdir( ) is called then it returns all the files and directory from the specified path.
- os.path.isfile( ) is called to filter that list and it only prints files not directories.
- You can see output below, here only files are printed.
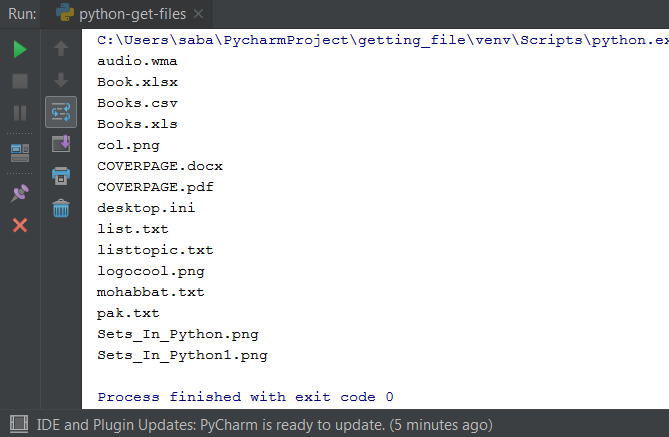
Listing Sub-directories
Now to list sub-directories, you have to write following program.
1 2 3 4 5 6 7 8 9 10 |
import os # Open a file path = r"C:\Users\saba\Documents" for files in os.listdir(path): if os.path.isdir(os.path.join(path, files)): print(files) |
- os.path.isdir( ) to confirm that a given path points to a directory that means it returns true if path is an existing directory.
Let’s check the output –
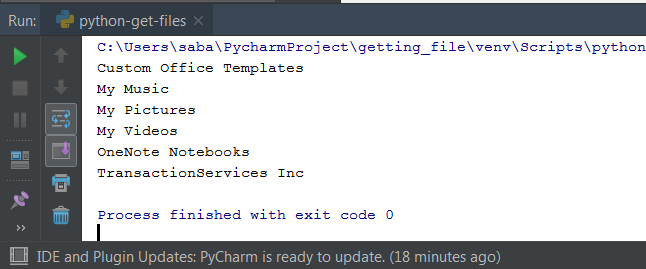
Here you can see only sub-directories are listed.
Python Get Files In Directory – Getting Files With Pathlib Module
In this section, you will learn directory listing using pathlib module.
- pathlib module offers classes representing filesystem paths with semantics appropriate for different operating systems.
- Python 3.2 or later is recommended, but pathlib is also usable with Python 2.7 and 2.6.
- So let’s see how can we do directory listing using pathlib module.
Directory Listing
Write the following code for directory listing using pathlib module.
1 2 3 4 5 6 7 |
from pathlib import Path path = Path(r'C:\Users\saba\Documents') for entry in path.iterdir(): print(entry.name) |
- First of all you have to import path class from pathlib module.
- Then you have to create a path object that will return either PosixPath or WindowsPath objects depending on the operating system.
- path.iterdir( ) return the path points to a directory, yield path objects of the directory contents. It is used to get a list of all files and directories of specified directory.
- Then simply print the entry name.
Now check the output, let’s see what will it show.
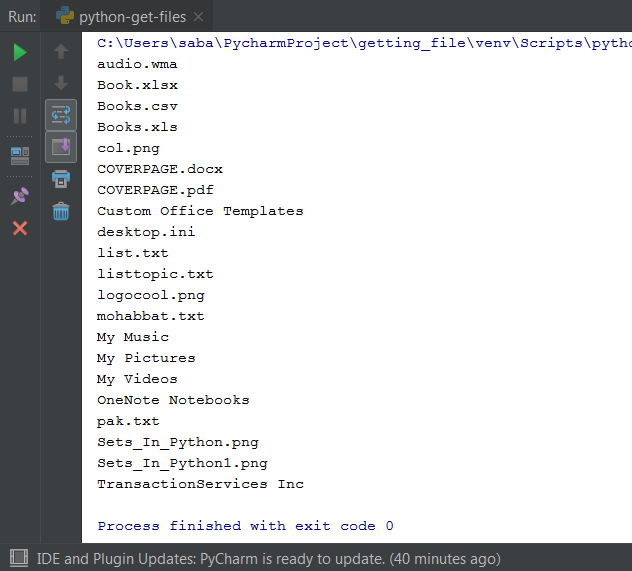
Listing all Files In A Directory
Now let’s see how to list all files in a directory using pathlib module.
1 2 3 4 5 6 7 8 9 |
from pathlib import Path path = Path(r"C:\Users\saba\Documents") files_in_path = path.iterdir() for item in files_in_path: if item.is_file(): print(item.name) |
- First of all call iterdir( ) method to get all the files and directories from the specified path.
- Then start a loop and get all files using is_file( ) method. is_file( ) return True if the path points to a regular file, False if it points to another kind of file.
- Then print all the files.
Now its time to check its output.
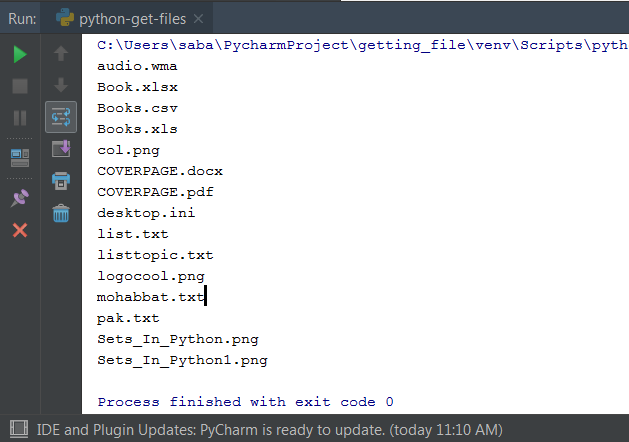
All files are listed successfully.
Listing Sub-directories
Write the following code to list subdirectories.
1 2 3 4 5 6 7 8 |
from pathlib import Path path = Path(r"C:\Users\saba\Documents") for dirs in path.iterdir(): if dirs.is_dir(): print(dirs.name) |
- is_dir( ) is called to checks if an entry is a file or a directory, on each entry of the path iterator.
- If it return True then the directory name is printed to the screen.
- Now check the output.
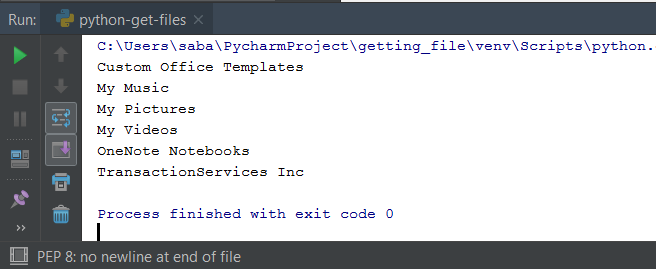
Conclusion
In this tutorial, you have seen various ways of directory listing in python. OS and pathlib module is very useful in listing files. You have also seen many methods like listdir( ), scandir( ) and iterdir( ) that helps in getting files in directory.
So i am wrapping Python Get Files In Directory Tutorial here. I hope, you found very helpful informations about getting file in directory using python. But anyway, if you have any query then your queries are most welcome. I will try to solve out your issues. So just stay tuned with Simplified Python and enhance your knowledge of python. Thanks everyone.