Dear python learners, in the last tutorial you have learned Convert Python To exe Tutorial . In this tutorial you will learn how to implement switch case statement in python. So welcome to Python Switch Case Statement Tutorial.
Switch case is a very powerful and important decision-making construct that are commonly used in modular programming. When you don’t want a conditional block cluttered with multiple if conditions, then, switch case can give a cleaner way to implement control flow in your program.
Contents
So let’s get ahead and see how can we implement switch case statement in python.
Python Switch Case Statement Tutorial – Getting Started
Unlike other programming languages like C, C++, java etc python does not have a switch case construct over the self. But don’t worry there are many other constructs like dictionary, lambda function and classes are available to write switch case statement in python. PEP 3103 will explain you why python have not switch case statement.
A Typical Switch Case Statement in C++
Firstly, we will see how a switch case statement looks like in another languages. So take a look of C++ switch case statement.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
string area(int i){ switch(i){ case 0: return “Cylinder” break; case 1: return “Square” break; case 2: return “Rectangle” break; case 3: return “Triangle” break; default: return “Invalid area” } } |
But as we know Python does not have this. So, achieving this, we use Python’s built-in dictionary construct to implement cases and decided what to do when a case is met. We can also specify what to do when none is met.
Have you checked best python IDEs for Windows
Python Switch Case Statement Implementation – Dictionary Mapping, if-elif-else ladder, Classes
Now we will discuss about 3 simple ways of implementing python switch case statement. So let’s see it one-by-one.
Python Switch Case Statement Using Dictionary Mapping
First of all we will see how to implement python switch case statement using Dictionary mapping. So let’s see how to do that.
Now write the below code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
def switch(): r = int(input("Enter Radius : ")) # it takes user input h = int(input("Enter Height : ")) # it takes user input # This will guide the user to choose option print("Press 1 for Surface Area\n press 2 for Literal Area \n press 3 for Volume ") # This will take option from user option = int(input(" your option : ")) # Calculate Surface Area Of Cylinder def Sar(): result = 2*3.17*r*(r+h) print(" Surface Area Of Cylinder = ",result) # Calculate Literal Area Of Cylinder def Lar(): result = 2 * 3.17 * r * h print("Literal Area Of Cylinder = ", result) # Calculate Volume Of Cylinder def vol(): result = 3.17*r*r*h print("Volume Of Cylinder = ", result) # If user enters invalid option then this method will be called def default(): print("Incorrect option") # Dictionary Mapping dict = { 1 : Sar, 2 : Lar, 3 : vol, } dict.get(option,default)() # get() method returns the function matching the argument switch() # Call switch() method |
Explanation
So now we will discuss the above code step by step.
- First of all you have to declare a switch method.
- In switch( ) method what you have to perform is that you have to take radius and height as an input from the user and perform operation on it according to user’s selection.
- So now declare first variable i.e., radius and second variable i.e., height.
- Then show a message for choosing option.
- Now take option input from the user.
- Now define sub method for calculating surface area, literal area and volume of cylinder.
- Then you have to create a dictionary that may be any name. And inside this, map the dictionary that means if user press option 1 then Sar( ) method will be performed, and if user press option 2 then Lar( ) method will be performed and so on.
- Now you have to get option value so that you can perform action according to it. So call the get() method on the dictionary object which returns the function matching the argument and invokes it simultaneously.
- And at last call the switch( ) method.
So now let’s check the output –
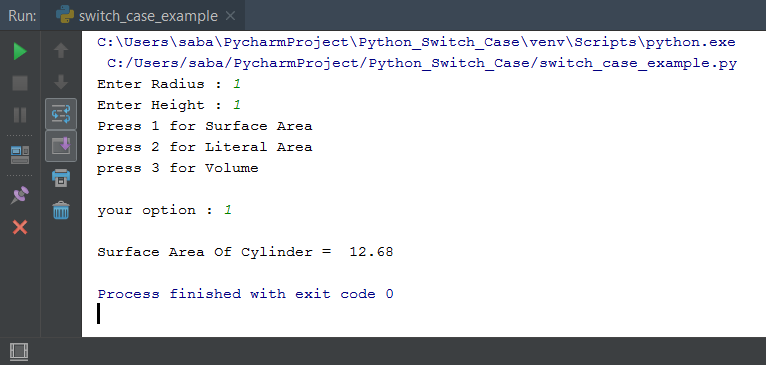
So guys we have successfully construct python switch case statement using dictionary. Now move to another method.
Let’s check Best python books for beginners
Python Switch Case Statement Using if-elif-else ladder
Constructing python switch case statement using if-elif-else ladder is very much similar as the dictionary mapping. But here we don’t need to define sub methods and dictionary, instead we have to create if-elif-else construct. It works as a switch construct in python.
So let’s see it practically. Write the following code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
def switch(): r = int(input("Enter Radius : ")) h = int(input("Enter Height : ")) print("Press 1 for Surface Area \npress 2 for Literal Area \npress 3 for Volume \n") option = int(input("your option : ")) if option == 1: result = 2*3.17*r*(r+h) print("\nSurface Area Of Cylinder = ",result) elif option == 2: result = 2 * 3.17 * r * h print("Literal Area Of Cylinder = ", result) elif option == 3: result = 3.17*r*r*h print("Volume Of Cylinder = ", result) else: print("Incorrect option") switch() |
- In this code above few lines are same as of the dictionary mapping so i am not explaining them here.
- Here the most important thing is that you have to declare if-elif-else ladder.
- So if the option is 1 then you have to calculate surface area of cylinder, and if the option is 2 then you have to calculate literal surface area, and if the option is 3 then you have to calculate volume, and if the option is not 1,2 or 3 then it will print incorrect option.
Now its time to check the output, so let’s see.
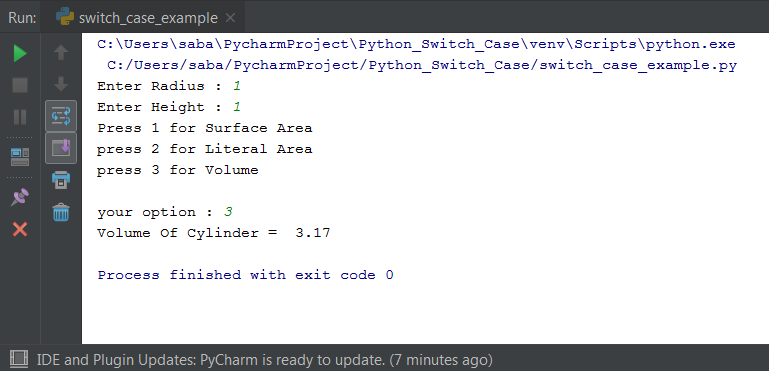
Python Calculator – Create A Simple GUI Calculator Using Tkinter
Python Switch Case Statement Using Class
So now we will see how to construct a switch case statement in python using class. So let’s start.
Write the following code –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
class PythonSwitchStatement: def switch(self, month): default = "Incorrect month" return getattr(self, 'case_' + str(month), lambda: default)() def case_1(self): return "January" def case_2(self): return "February" def case_3(self): return "March" def case_4(self): return "April" def case_5(self): return "May" def case_6(self): return "June" s = PythonSwitchStatement() print(s.switch(1)) print(s.switch(3)) print(s.switch(9)) |
Explanation
Now we will discuss what we have done in the above program.
- At first we have created a class named PythonSwitchStatement which defines switch( ) method. It also defines some functions specific to different cases.
- Switch( ) method takes the month as an argument, converts it to string and appends to the ‘case_’ literal. After that, the resultant string gets passed to the getattr() method.
- The getattr( ) method returns a matching function available in the class.
- And if the string doesn’t find a match, then the getattr() returns the lambda function as default.
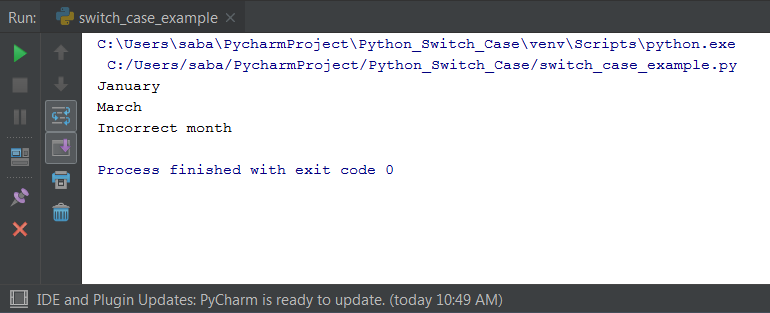
So guys this is all about implementation of switch case statement in python.
Conclusion
Although python does not have an in-built switch-case construct, but we can construct it using dictionary mapping, class and if-elif-else ladder. So i am wrapping Python Switch Case Statement Tutorial here. I hope you have enjoyed this tutorial, so if you have any query regarding this then feel free to comment. Surely i will solve your problems, till then stay tuned with Simplified Python.