So, here is Python CSV Reader Tutorial. It might be handy when you want to work with spreadsheets. Like you need to export or import spreadsheets. And the best thing is Python has the inbuilt functionality to work with CSVs. And you can do it very quickly.
Contents
What is CSV?
CSV stands for Comma Separated Values. It is like a spreadsheet but it stores data in plain text, and the values are separated by a comma (,).
We can easily represent the Tabular data in a CSV format.
Consider the following table.
Name | Rank | City |
Parmanu | 1 | Delhi |
Super Commando Dhruva | 2 | Rajnagar |
Doga | 3 | Mumbai |
The above table can be represented in CSV as
1 2 3 4 5 6 |
Name,Rank,City Parmanu,1,Delhi Super Commando Dhruva,2,Rajnagar Doga,3,Mumbai |
The above examples are showing a minimal CSV data, but in real world, we use CSV for large datasets with large number of variables.
Python CSV Module
We have an inbuilt module named CSV in python. We can use it to read or write CSV files.
So if you want to work with CSV, you have to import this module.
1 2 3 |
import csv |
Then you can work with CSV quickly, with the predefined functions. In this module, we already have all the necessary methods.
CSV Functions
We have many functions in the CSV modules, some of them are:
- csv.reader(csvfile, dialect=’excel’, **fmtparams)
- csv.writer(csvfile, dialect=’excel’, **fmtparams)
- csv.register_dialect(name[, dialect[, **fmtparams]])
- csv.unregister_dialect(name)
- csv.get_dialect(name)
- csv.list_dialects()
- csv.field_size_limit([new_limit])
But in this post, we will see how to read and write CSV files in our Python Project.
Python CSV Reader and Writer Project
Now lets, use the functions in our Python Project. Here I will be using PyCharm. I would recommend this IDE as it is the best according to me.
Check Best Python IDEs for Windows
But you can use any other IDE or source code editor.
Creating a new Project
- So in your PyCharm (I am using the community edition which is free), create a new Project.
- So I have a project named, CSVReader (Though we will be doing the Write Operation as Well 😛 ).
Python CSV Reader
- In your project create a new Python File named CSVReader.py.
- Now, first we will import the csv module in the file that we just created.
1 2 3 |
import csv |
- But to read csv, the first thing we need is a csv file itself. So in your project create a new file named data.csv and write the following data in it.
1 2 3 4 5 6 |
Name,Rank,City Parmanu,1,Delhi Super Commando Dhruva,2,Rajnagar Doga,3,Mumbai |
- So, we have a CSV file to read. Now we will read the contents of this file, so open the python file CSVReader.py that we created.
1 2 3 4 5 6 7 8 9 |
import csv with open("data.csv", 'r') as csvfile: reader = csv.reader(csvfile) for row in reader: print(row) |
What we Did?
Here first we are opening the file that contains the CSV data (data.csv), then we created the reader using the reader() function of csv module. Then we are traversing through all the rows using the for loop, and we are printing the row as well.
You can run the code to see the output.
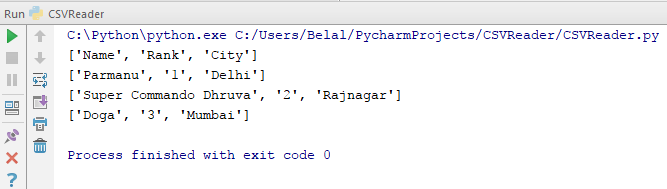
You see we are getting the rows, but it is same as reading a normal file. As it is a CSV data we can read the data separately as well.
Modify the code as below.Â
1 2 3 4 5 6 7 8 9 10 11 12 |
import csv with open("data.csv", 'r') as csvfile: reader = csv.reader(csvfile) for row in reader: print(row[0], end='\t\t') print(row[1], end='\t\t') print(row[2], end='\n') print('--------------------------------') |
Running the above code will give you the output as.
But don’t forget to close the opened file as.
1 2 3 |
csvfile.close() |
Python CSV Writer
Now lets see how we can write CSV files.
- We need to create a new file. I have created CSVWriter.py in the project and write the following code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
row = input("Enter how many rows you want to insert?") print(row) with open("mydata.csv", "w") as csvfile: line = "" for i in range(0, int(row)): line += input("Enter name:\t") + "," line += input("Enter rank:\t") + "," line += input("Enter city:\t") + "," csvfile.writelines(line) print("File written successfully") |
- Running the above code will give you the following output.
- Yeah, it is working absolutely fine.
That’s all for this Python CSV Reader Tutorial friends. If you have any question, then leave your comments. If you found this tutorial helpful, then please SHARE it with your friends. Thank You 🙂