Hi friends, here is another useful post where I will show you a simple Python Rest API Example. In this tutorial we will learn about what is REST API and how to build it in python.So let’s start the tutorial.First of all we will learn what is REST API.
What is REST API
- It stands for Representational State Transfer.
- Roy Fielding defined REST API in 2000.
- It is an architectural design that have many constraints for designing web applications.
Architectural Constraints Of REST API
- Client-Server Architecture – Client is responsible for handling front-end and server for back-end.They work separately without depending to each other.
- Statelessness – It means server will not store any information about the latest HTTP request that the client has sended.Therefore each request is treated as new request every time.
- Cacheability – It saves times and improve scalability and performance.
- Layered system – It means that , there may be intermediary things like Proxies,Gateways and another REST APIs between the client and the server.
- Uniform Interface – The interaction of all components in a REST API will have the same interface. It will have the same set of protocols, uniform resource identifiers (URI), methods, and representations.
Real world concept of REST API
- Furthermore we can understand the concept of REST API clearly in addition to take an example.
- So take an example from real world . Let’s consider we are going to book flight tickets.Hence there is two method for booking. First one is to book tickets directly from flight’s website and second one is via some travel agencies.
- In case of travel agencies rather to flight’s websites , they keep records about different flights simultaneously .They are connected with the database of all flights.
- Booking through travel agencies, we need not go to websites of several flights because they keeps records of all flights in one place.
- Hence the travel agencies play the role as a REST API.
- So i hope REST API concept is cleared to all of you.
Real world example of REST APIs
- Gmail
- GitHub
- Weather Underground etc
RESTful web services
Consequently Designing of REST API is based on HTTP protocol. It also provides some methods such as –
- GET – to read data from database
- POST – to insert new data in database
- PUT – to update data in database
- DELETE – to delete data from database
HTTP Status Codes
Whenever client make request to the server , server send status code as a result i.e., response to the client.In addition Some of Status Codes are following –
Status code | Meaning |
100 | continue |
102 | processing |
200 | ok |
201 | created |
202 | accepted |
302 | found |
304 | not modified |
403 | forbidden |
404 | not found |
503 | Service unavailable |
Building our Python Rest API Example
So, now we will start building REST API in Python. Here I am using BOTTLE framework rather than any others because of its simplicity. Before proceeding to coding. First of all we will understand what is BOTTLE framework.
BOTTLE Framework
In this tutorial, i am using BOTTLE framework because it is especially relevant.So the first question is – what is BOTTLE .
- BOTTLE is nothing but a simple, fast and light weight micro web framework for python programming language.
- Most python standard library have many dependencies but BOTTLE has no dependencies. It is distributed as a single file module.
- it helps in developing web applications easily and fastly.
Overview
Now start to building Rest Api.
- First we create a dictionary that stores informations about employees.
- Implement get service for displaying all the employee’s informations.
- Implement get service for displaying only a particular employee’s information according to name.
- create post service for adding information in employee’s database.
- create delete service for deleting employee’s informations.
Stuffs Required
- PyCharm IDE (i preferred PyCharm but you can use anyone)
- BOTTLE framework
- POSTMAN REST client for testing
- knowledge of python
Let the Code Begin
First of all create a project and name it. Create a python file inside the project. I hope you know about creating project.
Installing BOTTLE module
Go to File menu and click on Setting, as a result a window appears like below.
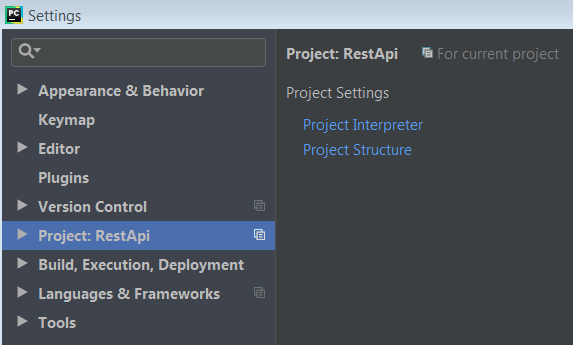
Furthermore expand Project:RestApi option and select Project Interpreter.As a result a window will appear.Then search the bottle module and click on Install Package.
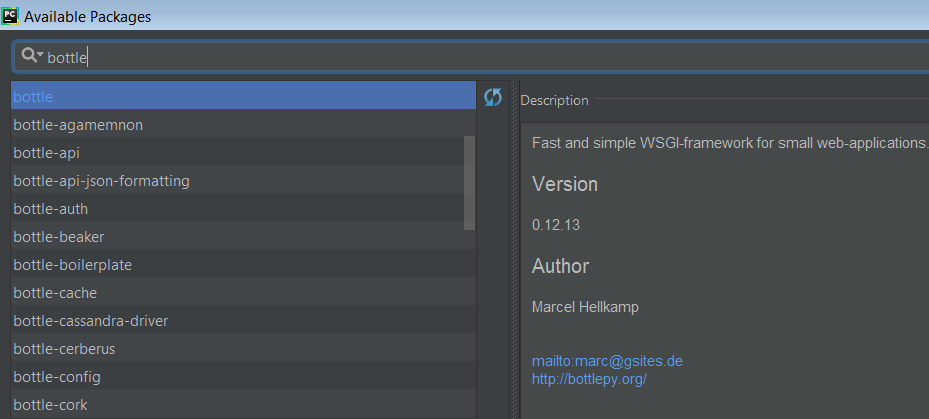
Finally Bottle has been successfully installed.
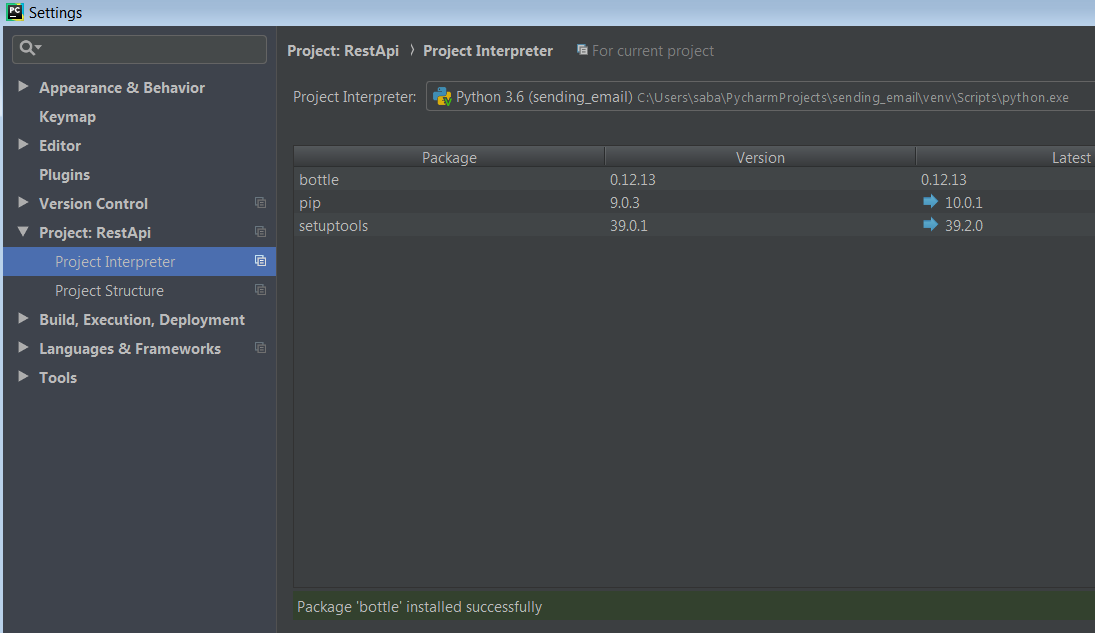
Importing Bottle
1 2 3 4 |
from bottle import run run(reloader=True, debug=True) |
Bottle library have run() method.This method starts a server instance.Here run() method contain two parameters:
reloader : whenever we changes in our code we have to restart the server.Reloader start auto-reloading server.It’s default value is False.
debug : It is very helpful in early development.It provides a complete informations whenever an error occurred.
Creating a dictionary
Here i am creating a dictionary that stores informations of employees.
1 2 3 4 5 |
employee = [{'name' : 'Sam','address':'Ranchi','Dept':'HR'}, {'name': 'Sarah', 'address': 'Ranchi', 'Dept': 'MGR'}, {'name': 'Arsh', 'address': 'Delhi', 'Dept': 'HR'}] |
So the Dictionary name is employee. It have three items name, address and Dept.I am taking details of three employees but you can take more than that.
Get All Employee Details
first we need to import get service.
1 2 3 |
from bottle import run,get |
It means any get request will come in between will be passes in the function underneath the decorator.
1 2 3 4 5 |
@get('/employee') def getAllEmployee(): return{'employees':employee} |
- Create a @get() decorator.
- Decorator is any python object for modifying a function or a class.
- Furthermore for a huge list of decorators check the link Python Decorator Library
- we passes a null point called employee.
- Then call a function getAllEmployee() .And simply returns the dictionary with a key employees and value employee.
- This will return all the items stored in dictionary.The complete code is –
1 2 3 4 5 6 7 8 9 |
from bottle import run, get employee = [{'name' : 'Sam','address':'Ranchi','Dept':'HR'}, {'name': 'Sarah', 'address': 'Ranchi', 'Dept': 'MGR'}, {'name': 'Arsh', 'address': 'Delhi', 'Dept': 'HR'}] @get('/employee') def getAllEmployee(): return{'employees':employee} |
Testing the API
Hence On running above code we get an url like below.

Here i am using POSTMAN REST client for testing API. This is POSTMAN screen.
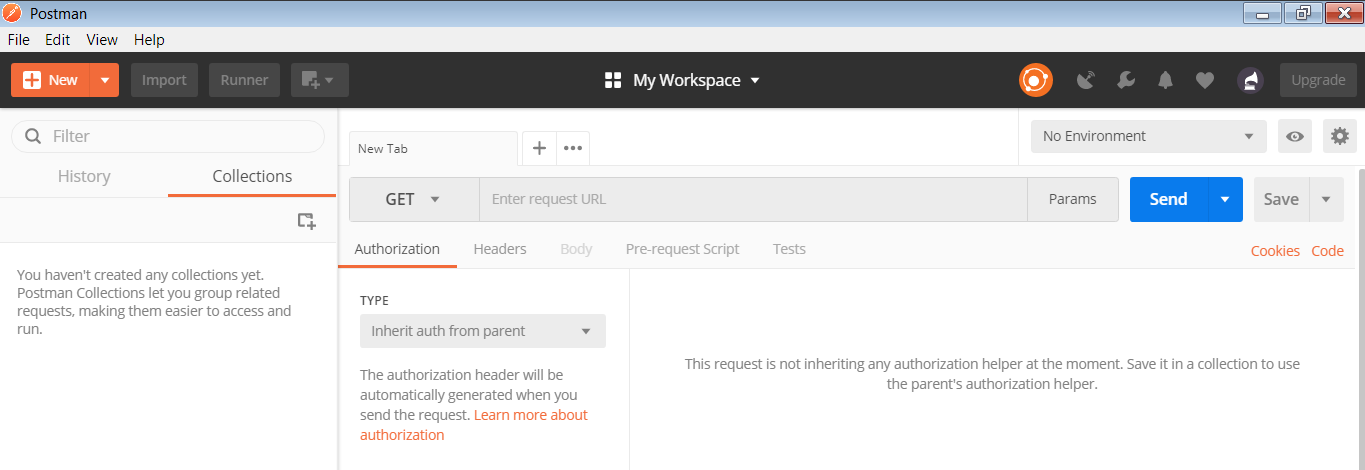
Now type the URL and click send button, we get following output.
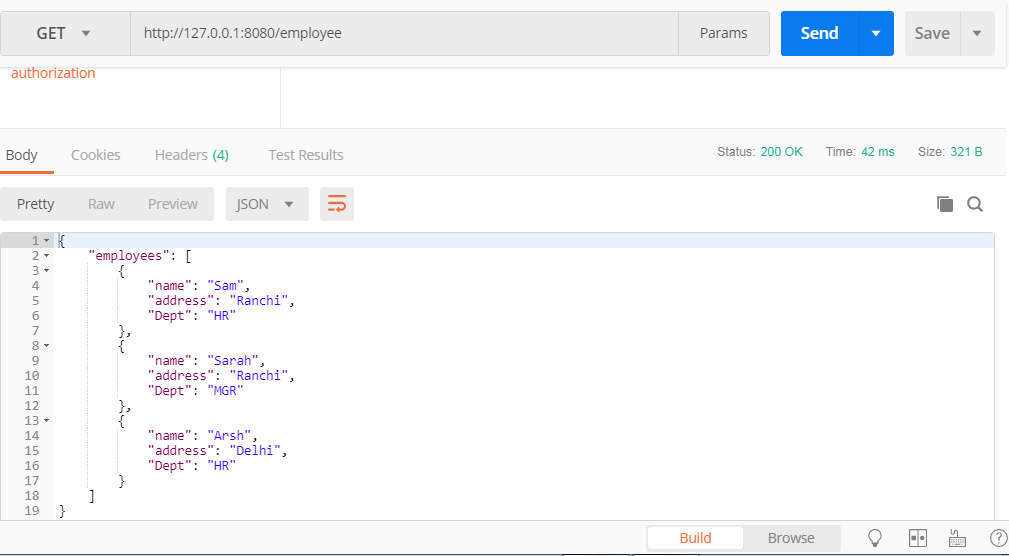
Get a particular employee details
We can also get a particular employee’s details rather than to get all employees.Here i am doing so according to employee’s name.
1 2 3 4 5 6 |
@get('/employee/<name>') def get_Emp_by_name(name): the_emp = [emp for emp in employee if emp['name'] == name] return{'employee' : the_emp[0]} |
- Again take a decorator and pass a variable name.
- Define a method get_Emp_by_name(name). It takes the parameter what the variable passed to the decorator .
- Create a variable that search the dictionary by name.
- Return the selected employee.
Now run the application.Pass the url along with employee name. It will display the details of passed employee.
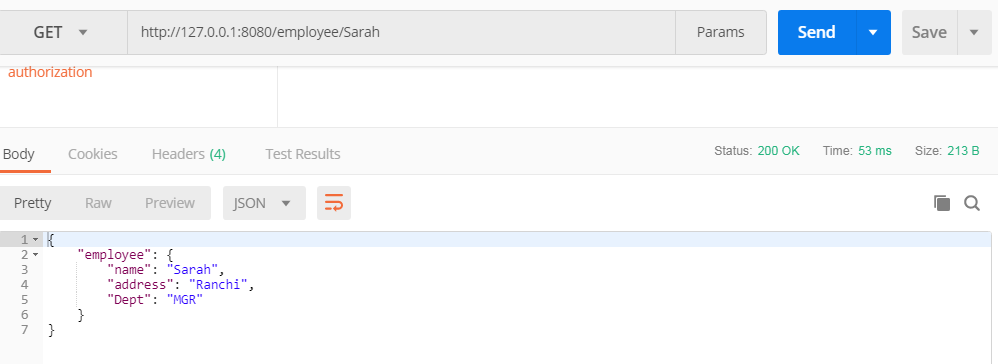
Add the employee
Furthermore i am going to add an element. It is pretty simple.first of all import the post and request method.
1 2 3 |
from bottle import run, get, post ,request |
1 2 3 4 5 6 7 |
@post('/employee') def add_emp(): new_emp = {'name' : request.json.get('name'),'address' : request.json.get('address'),'Dept':request.json.get('Dept')} employee.append(new_emp) return {'employees' : employee} |
- Create a post decorator .
- Call a function add_emp(). This function is going to take JSON object with the information given in dictionary.
- Create a variable new_emp to add the employee details.
- request is a HTTP library .It allows us to send HTTP/1.1 requests using Python.It is used to add data via simple Python libraries
- append() method add the new employee in existing list.
Now run the code. Insert url , select post from dropdown. Click on Body select raw and in text select JSON(application/json).Insert the employee’s details as follows –

On clicking send button we will get the following output as a result.
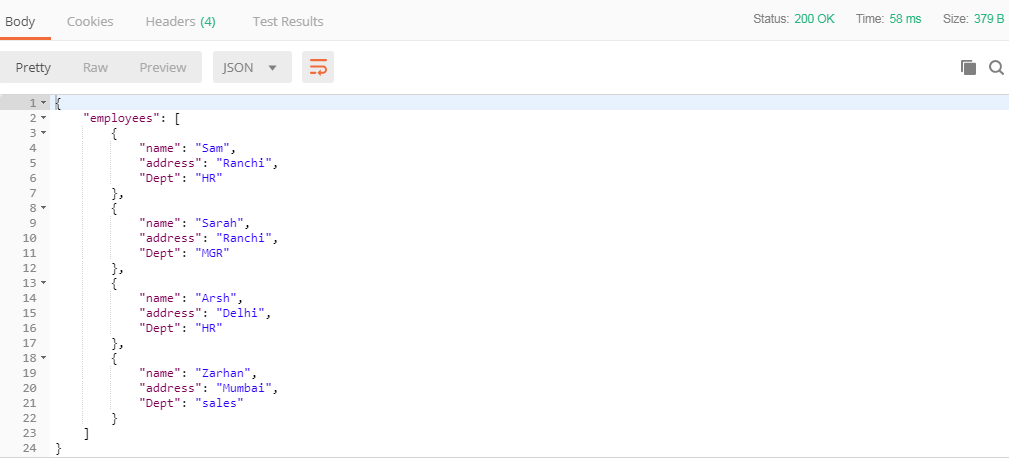
We can see the new data is added to the existing employee’s list.
Deleting the employee details
Hence the last thing is to delete employee.For this write the following code.First import the delete library.
1 2 3 |
from bottle import run, get, post ,request,delete |
Now create a delete decorator. The code is seems like the code of getting a particular employee.But it have a little bit change.
1 2 3 4 5 6 7 |
@delete('/employee/<name>') def remove_emp(name): the_emp = [emp for emp in employee if emp['name'] == name] employee.remove(the_emp[0]) return {'employees': employee} |
- Call a method remove_emp() and pass the parameter name i.e., employee name.
- create a variable for searching the selected element in dictionary .
- remove() method delete the selected object from the employee list.
- return the updated list of employee.
Hence run the code.Select delete from dropdown. Enter url along with employee name(i am taking employee Arsh ) and click send button.The output will be as follows –
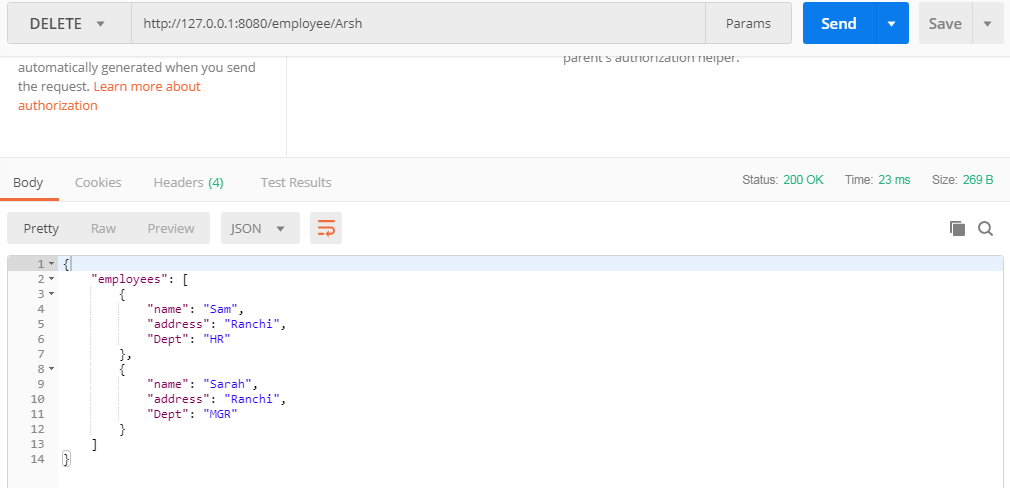
Finally the employee Arsh is successfully removed from the employee list.
So this is a tutorial of Building REST API in Python . In conclusion, this is a helpful post for creating REST API . If you have any query regarding to this post you can comment freely. Thank You.