Welcome to Python Run Shell Command On Windows tutorial. In this tutorial, you will learn, how to run shell command in python. So let’s move forward.
Python Run Shell Command On Windows
What is Shell ?
- In computer science shell is generally seen as a piece of software that provides an interface for a user to some other software or the operating system.
- So the shell can be an interface between the operating system and the services of the kernel of this operating system
Python Modules For Running Shell command
Python provides lots of modules for executing different operations related to operating system.
Generally there are two important modules which are used to run shell command in python.
- Subprocess module
- OS module
Python Run Shell Command Using Subprocess module
The subprocess module allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This module intends to replace several older modules and functions:
- os.system
- os.spawn*
- os.popen*
- popen2.*
- commands.*
The subprocess module allows users to communicate from their Python script to a terminal like bash or cmd.exe.
Now we will see different functions of subprocess module.
subprocess.call()
call() method create a separate process and run provided command in this process.
Write the following code to implement call() method of subprocess module.
1 2 3 4 |
import subprocess subprocess.call('dir', shell=True) |
In this example, we will create a process for dir command
It’s output will be as follows.
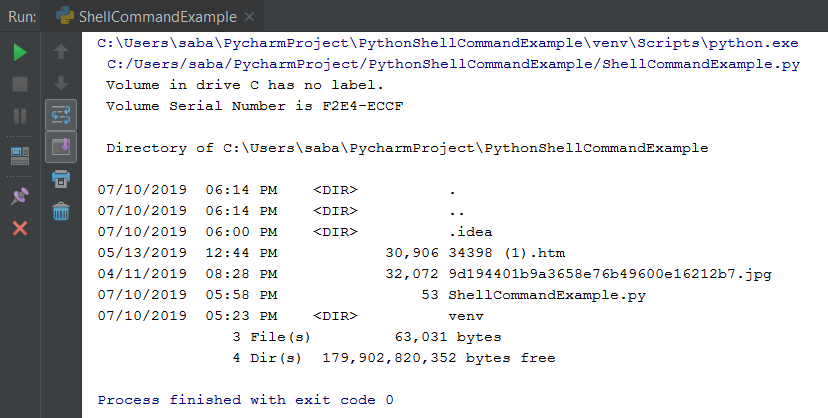
subprocess.check_output()
check_output()is used to capture the output for later processing. So let’s see how it works.
1 2 3 4 5 |
import subprocess output = subprocess.check_output('dir', shell=True) print(output) |
Output
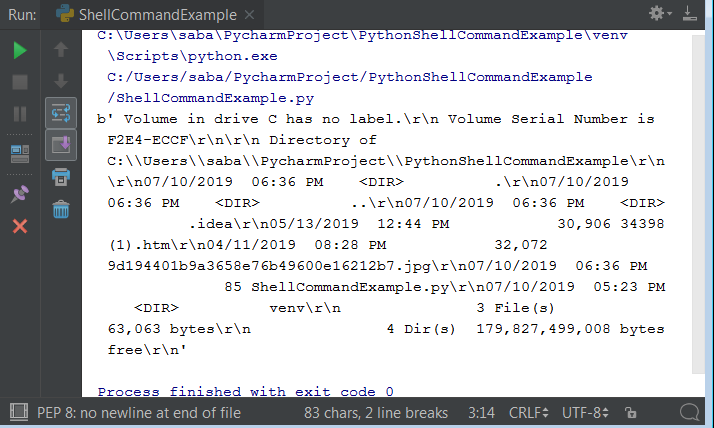
Python Run Shell Command Using OS module
The OS module is used to interact with underlying operating system in several different ways.
OS is an python built-in module so we don’t need to install any third party module. The os module allows platform independent programming by providing abstract methods.
Executing Shell scripts with os.system()
The most straight forward approach to run a shell command is by using os.system().
1 2 3 4 |
import os os.system('dir') |
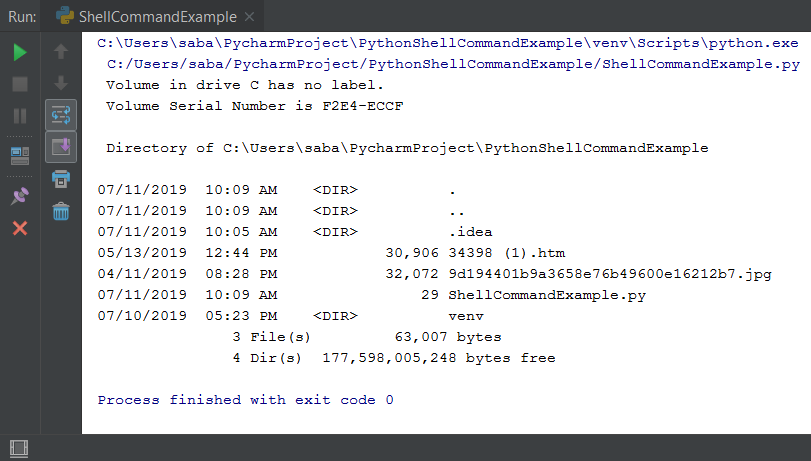
Capturing Output
The problem with this approach is in its inflexibility since you can’t even get the resulting output as a variable. os.system() doesn’t return the result of the called shell commands. So if you want to capture output then you have to use os.popen() method.
The os.popen() command opens a pipe from or to the command line. This means that we can access the stream within Python. This is useful since you can now get the output as a variable. Now let’s see it practically, so write the following code.
1 2 3 4 5 |
import os output = os.popen('dir').readlines() print(output) |
- Here i used readlines() function, which splits each line (including a trailing \n).
- You can also use read() method which will get the whole output as one string.
Now it’s output will be as follows.
So guys, that’s it for Python Run Shell Command On Windows tutorial. I hope it is helpful for you and if you have any query regarding this post then ask your questions in comment section. Thanks Everyone.
Related Articles :
- How To Add Code To GitHub Using PyCharm
- Sets In Python Tutorial For Beginners
- Join Two Lists Python – Learn Joining Two Lists With Examples