Hi everyone, in this Python Split String By Character tutorial, we will learn about how to split a string in python. Splitting string means breaking a given string into list of strings. Splitting string is a very common operation, especially in text based environment like – World Wide Web or operating in a text file. Python provides some string method for splitting strings. Let’s took a look how they works.
Python Split String By Character Tutorial – Getting Started With Examples
When splitting string, it takes one string and break it into two or more lists of substrings. Some time we may need to break a large string into smaller strings. For splitting string python provides a function called split().
What Is split() function ?
- split() method breaks a given string by a specified separator and return a list of strings.
Syntax
1 2 3 |
string.split(separator, maxsplit) |
- Separator is a delimiter at which the string splits. If the separator is not specified then the separator will be any whitespace. This is optional.
- Maxsplit is the maximum number of splits.The default value of maxsplit is -1, meaning, no limit on the number of splits. This is optional.
Splitting String By Whitespace
If we don’t specify any separator split() method takes any whitespace as separator.
Let’s understand it with an example.
1 2 3 4 5 6 7 |
Mystring = 'Learn splitting string' splitted_string = Mystring.split() print('Splitted String is : ', splitted_string) |
- This code will split string at whitespace.
So the output of this code is –
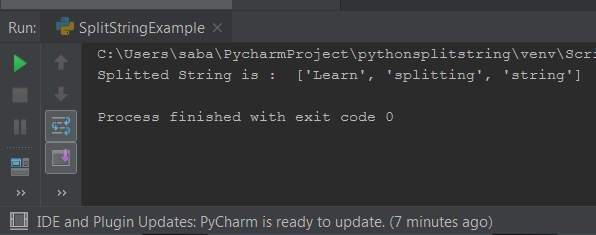
In the output, you can see that the string is broken up into a list of strings.
Splitting String By Colon ‘ : ‘
Now we will use : as a separator. Let’s see what happend.
1 2 3 4 5 6 7 |
Mystring = 'Welcome:To:Simplifiedpython' splitted_string = Mystring.split(':') print('Splitted String is : ', splitted_string) |
- This code will split string at : .
And the output is –
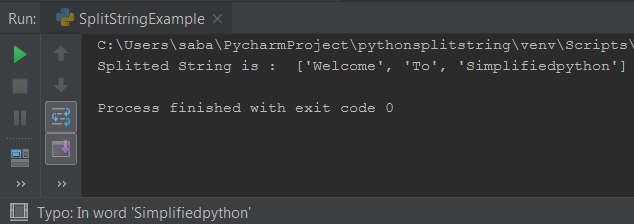
You can see that the colon(:) has been removed and string is splitted.
Splitting String By Hash Tag ‘ # ‘
Now we will use # as a separator, so for this the code is following.
1 2 3 4 5 6 7 |
url = "simplifiedpython.net#1000#2018-10-22" splitted_data = url.split("#") print('Splitting String By # :', splitted_data) |
- This code will split string at #.
Let’s see the result
All the # has been removed and string is splitted into list of substrings.
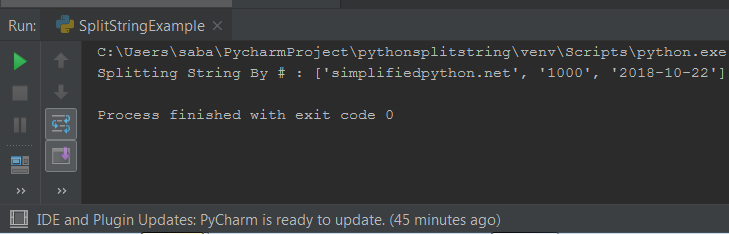
Splitting String By Comma ‘ , ‘
Now let’s see how to split string by comma. So write the following code.
1 2 3 4 5 6 7 |
Mystring = "Python,Is,Awesome" splitted_string = Mystring.split(",") print('Splitting String By , :', splitted_string) |
- This code will split string at , .
Result
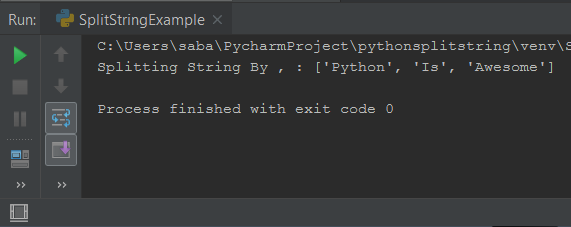
Splitting String By Position
Now if you want to split a string at particular position. For example string is “PythonIsProgrammingLanguage” , and you want to split this string at 4th position. So the code for this will be as follows –
1 2 3 4 5 6 7 8 |
Mystring = "PythonIsProgrammingLanguage" print('Original String :', Mystring) print('Splitting String By Position :', [Mystring[i:i+4] for i in range(0, len(Mystring), 4)]) |
- This code will split string at 4th position.
Result
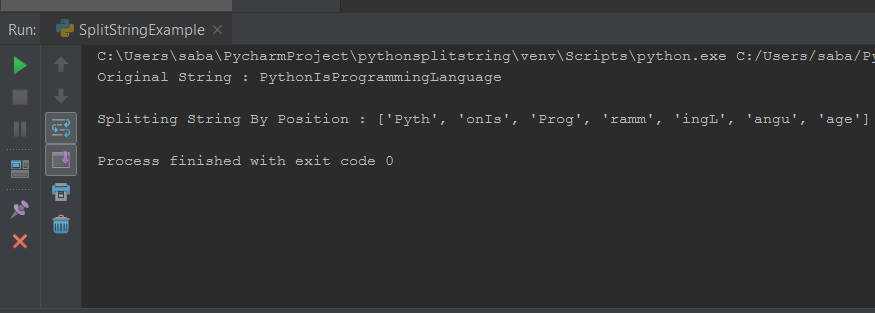
You can see the string is broken up from 4th position and splitted into list of substrings.
Splitting String By A Particular Characters
Now we will split string by a particular character. Let’s take an example “Python Is Programming Language”, and now you have to split this string at character P . So code for this is like below.
1 2 3 4 5 6 7 8 |
Mystring = "Python Is Programming Language" print('\nOriginal String :', Mystring,) print('\nSplitting String By Position :', Mystring.split('P')) |
- Here we have used P as a separator, so the result is as follows.
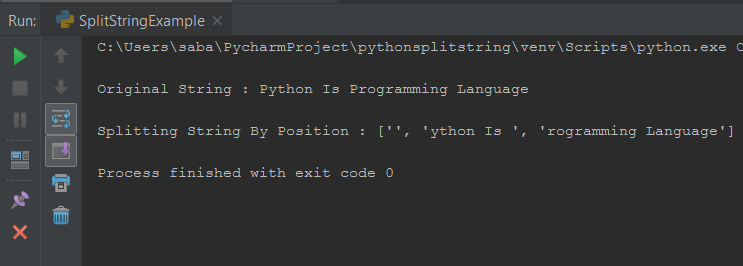
- All the P character has been removed from this string but whites paces are still in there because separator is a character.
Splitting String By Special Characters
Now we will see how to split string by special characters like \n, \t and so on. Let’s implement it in examples.
1 2 3 4 5 6 7 8 |
Mystring = "Python \t Is Programming Language" print('\nOriginal String :', Mystring,) print('\nSplitting String By Position :', Mystring.split('\t')) |
- Here i am taking a special character \t i.e., tab.
- This code will split string at \t .
- So see the result.
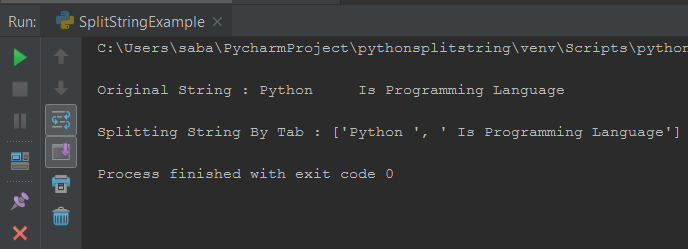
Splitting String By Maxsplit
Now we will see splitting string by maxsplit. It is a number, which tells us to split the string into maximum of provided number of times. So let’s move towards its implementation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Mystring = "Python Is Programming Language" print('\nOriginal String :', Mystring,) print('\nSplitting String By Maxsplit=4 :', Mystring.split(maxsplit=4)) print('\nSplitting String By Maxsplit=1 :', Mystring.split(maxsplit=1)) print('\nSplitting String By Maxsplit=0 :', Mystring.split(maxsplit=0)) |
Result
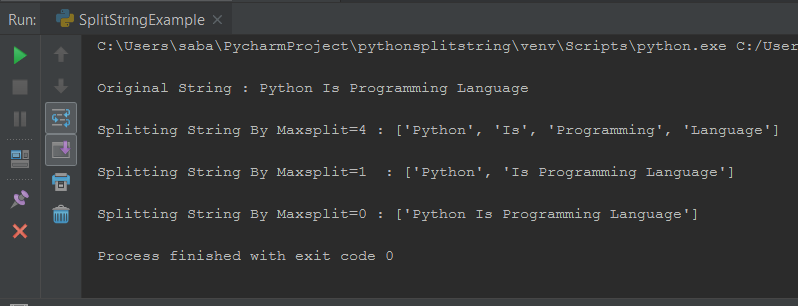
Now we will take an another example in which we will specify both separator as well as maxsplit.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Mystring = "Python#Is#Best#Suited#For#AI" print('\nOriginal String :', Mystring,) print('\nSplitting String By Maxsplit=4 :', Mystring.split('#', maxsplit=4)) print('\nSplitting String By Maxsplit=1 :', Mystring.split('#', maxsplit=1)) print('\nSplitting String By Maxsplit=0 :', Mystring.split('#', maxsplit=0)) |
And the output of this example is –
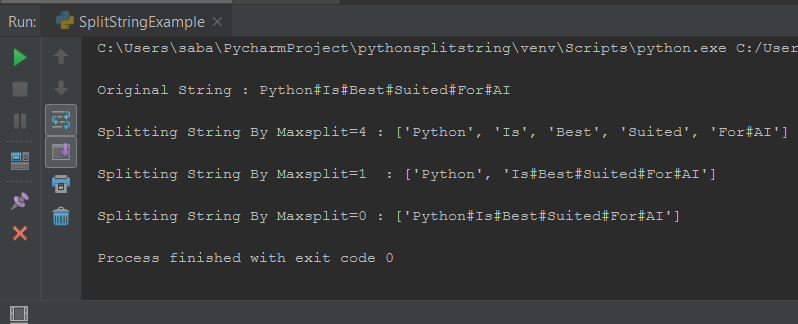
- We can see that when value of maxsplit is 4 then string is splitted into a list of substrings where first four string is splitted at # and remaining are same.
- When value of maxsplit is 1 then string is splitted into a list of substrings where only first string is splitted at # and remaining are same.
- When maxsplit is 0 then no splitting occur because the value is zero.
Recommended Posts
- Reverse A List Python – Reverse A List With Examples
- Join Two Lists Python – Learn Joining Two Lists With Examples
- Python Convert String To Datetime – Convert String To Datetime Using datetime Module
- Python Binary To Decimal Tutorial With Examples
- Sublime Run Python – Running Python Programs On Sublime Text 3
So friends, now i am wrapping up Python Split String By Character tutorial. If you have any query then please let me know this. And please share this post with your friends and suggest them to visit simplified python for best python tutorials. Thanks Everyone.